In this blog, we'll explore what React-Redux is, why do we need it, how it works, and how to
use it in your projects.
Redux is a state-management library for JavaScript applications. We can use it with React, Angular,
Vue, Vanilla JS. In this blog, we will discuss Redux in React.
React-Redux is a widespread library for managing states in React applications. Redux is a state-
container which provides predictable and scalable way to manage the state of your application,
making it convenient to write, test and maintain your code.
What is React-Redux?
React-Redux is a library that provides a way to manage the state of your React application. It is built
on top of Redux, which is a state management library for JavaScript applications. React-Redux
provides a set of tools and APIs that make it easier to connect your React components to your Redux
store, and to handle updates to the store in a predictable way.
Why do we need Redux?
While working on a React application with multiple components, often there’s a need to pass some
data to a child-component or two. Now to deliver that piece of data to the child component(s), it has
to pass through hierarchy of components who doesn’t need the data but still has to pass within
them so that the neighbouring child component(s) can access it.
This is where Redux comes into the picture. It provides a centralized space called store where data is
stored and can be accessed by any components in the React-app directly. Thus, Redux allows you to
manage your app's state in a single place and keep changes in your app more predictable and
traceable.
How Redux works?
React-Redux works by providing a set of higher-order components (HOCs) and hooks that you can
use to connect your components to your Redux store. These HOCs and hooks provide a way to
access the state and dispatch actions to update the state.
Redux institutes three primary objectives –
- What to do? //ACTION
- What to do? //REDUCER
- Object which holds the state of the application! //STORE
The main components of React-Redux are –
- Provider: This component provides the Redux store to your React application. It wraps your
entire application and passes the store down to all the child components. - useSelector(): This is a hook that you can use to select data from the Redux store.
- useDispatch(): This is a hook that you can use to dispatch actions to update the Redux store.
How to use React-Redux?
Here, I’m making a simple INCREMENT/DECREMENT counter using Redux. Let's go through the steps
to use React-Redux in your project.
Step 1: Install React-Redux
The first step is to install React-Redux in your project. You can do this using npm or yarn:

OR

Step 2: Make two folders named ‘actions’ and ‘reducers’ in /src and a JavaScript file named as
“store.js” as such-
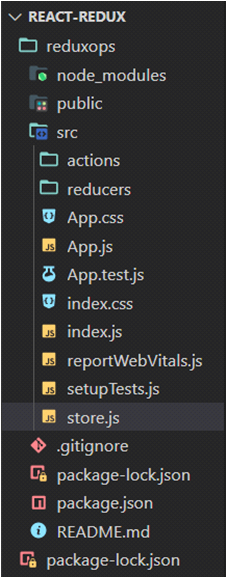
Step 3: Here we will define what actions are to be performed in our app. So, we will create an
“index.js” file inside ‘/src/actions’ and specify the actions as such-
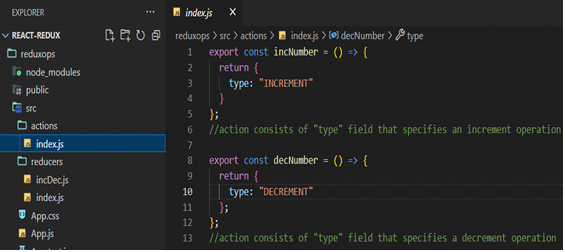
Step 4: Now, we will specify how to do the increment/decrement action which we’ve declared
earlier in /src/actions folder. So, we will create a file named “incDec.js” and an “index.js” inside
/src/reducers folder. Inside “incDec.js” we will assign the actual operation over the actions as such-
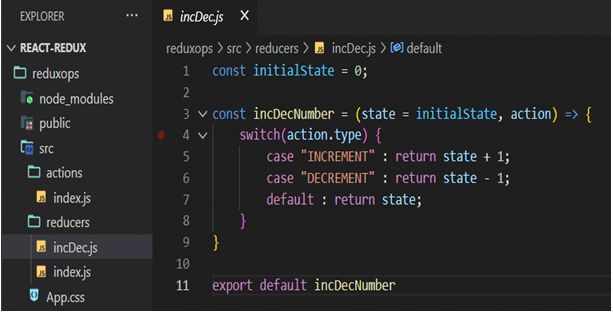
Step 5: Now, we will combine the reducers in /src/reducers/index.js as such-
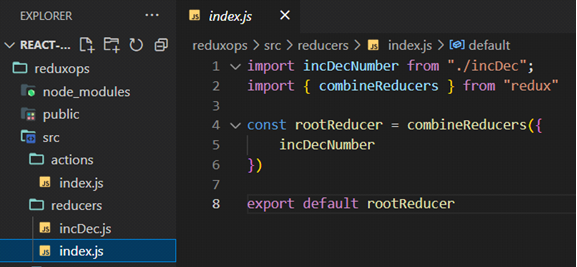
Step 6: Now, we will import rootReducer into our src/store.js file and also, we will create store in
store.js as such-
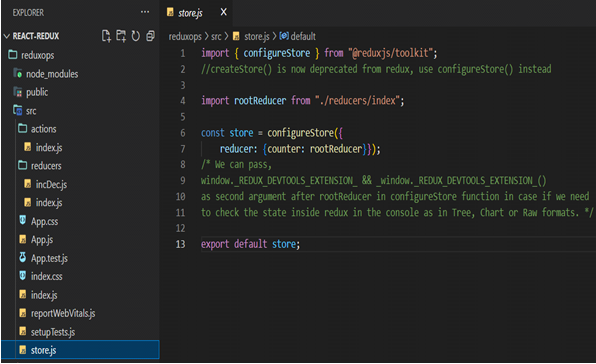
Step 7: Now, we will import store from ‘/src/store.js’ and Provider from ‘react-redux’ inside
‘/src/index.js’ and also, we’ll have to wrap our App component inside <Provider> and also pass store
as a prop to deliver data to the App component.
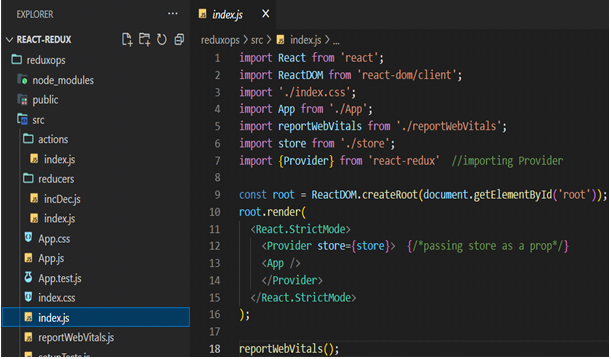
Step 8: Now to finally call the data and get the data in ‘/src/App.js’ , we’ll have to import useSelector
and useDispatcher from ‘react-redux’ and finally pass an arrow function over onClick event calling
dispatch() with parameter of Increment/Decrement as such-
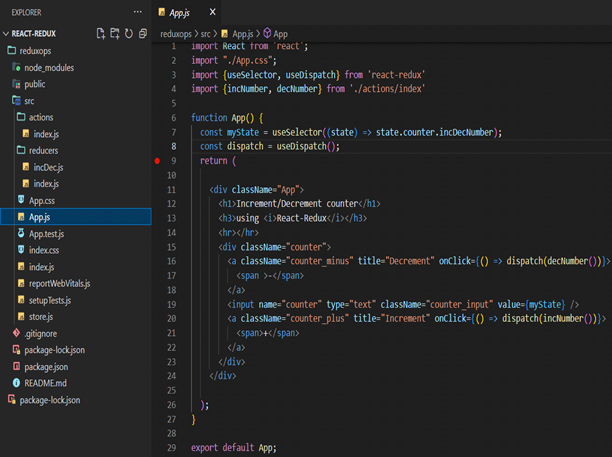
Finally, the UI should look something like –
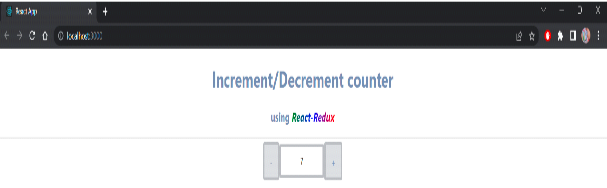
In conclusion, React-Redux is a powerful combination of two popular JavaScript libraries that allows
developers to build scalable and efficient web applications. React is a UI library that helps in building
reusable UI components while Redux is a state management library that provides a predictable state
container for JavaScript applications. The combination of these two libraries allows for the creation
of complex applications that can handle large amounts of data and complex UI interactions.
React-Redux simplifies the management of the application’s state by centralizing it in one place,
allowing for easy debugging and testing of the application. It also helps to prevent unnecessary re-
renders of components by selectively updating only the necessary parts of the UI.
React-Redux has become the go-to choice for many developers and companies for building scalable
and efficient web applications. Its popularity can be attributed to its simplicity, predictability, and
ease of use. With the constantly evolving JavaScript ecosystem, React-Redux continues to adapt and
improve, making it a powerful tool for building modern web applications.
Nice blog….very informative
2023-04-05 at 5:05 pm
Nice Blog………. Sagar
2023-04-05 at 5:17 pm