Django comes with a variety of command line utilities that can be either invoked using django-admin.py or the convenient manage.py script.
We can also add our own commands. Those management commands can be very handy when you need to interact with your application via command line using a terminal and it can also serve as an interface to execute cron jobs.
Introduction:
You are probably already familiar with commands like
python manage.py startapp
- python manage.py startproject
- python manage.py makemigrations
- python manage.py migrate
- python manage.py runserver
- python manage.py collectstatic
To see a complete list of commands you can run the command below:
python manage.py help
We can create our own commands for our apps and include them in the list by creating a management/commands directory inside an app directory, like below:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 |
mysite/ |-- core/ | |-- management/ | | +-- commands/ | | +--my_custom_command.py | |-- migrations/ | | +-- __init__.py | | -- __init__.py | | -- admin.py | | -- apps.py | | -- models.py | | -- tests.py | +-- views.py | -- mysite/ | | -- __init__.py | | -- settings.py | | --urls.py | | --wsgi.py +-- manage.py |
The name of the command file will be used to invoke using the command line utility.
For example, if our command was called my_custom_command.py , then we will be able to execute it via:
python manage.py my_custom_command
Basic Example
A basic example of what the custom command should look like:
management/commands/current_time.py
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 |
from django.core.management.base import BaseCommand from django.utils import timezone class Command(BaseCommand): help = ‘Displays current time’ def handle(self, *args, **kwargs): time= timezone.now().strftime(‘%X’) self.stdout.write(“It’s now %s” % time) |
Styling:
We can improve the output by setting an appropriate color to the output message.
self.stdout.write(self.style.SUCCESS(”It’s now %s” % time))
Basically a Django management command is composed by a class named command which inherits from BaseCommand.
The command code should be defined inside the handle() method.
See how we named our module current_time.py.
This command can be executed as:
python manage.py current_time
Output:
It’s now 06:35:31
Find More Blogs
PODMAN
Spread the love 1.Introduction Podman: The Modern Container
Running Your Java Program (JAR) 24/7 on AWS EC2 Automatically
Spread the love Introduction Running a Java application
Building a REST API with Spring Boot
Spread the love Introduction REST (Representational State
Custom API to Fetch Customer Data by Customer ID in Spryker
Spread the love Introduction In this blog post, we will
Creating a Custom Module for a Custom Frontend Page in Spryker
Spread the love To create a custom module for a custom
How to create custom module api get category list without access token in Spryker
Spread the love To create a custom API for fetching a
Creating a Custom Glue API in Spryker
Spread the love Spryker’s Glue API is a powerful tool
Get AI Generated 360-Degree View of Account Summary in Dynamics 365 Sales
Spread the love Navigating the demanding landscape of sales
Enhancing Productivity with Dynamics 365: The Power of Session Restore
Spread the love Enhancing Productivity with Dynamics 365:
Salesforce Admin
Spread the love In our last blog, we discussed common
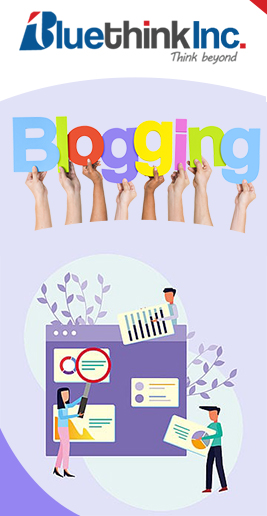
bluethinkinc_blog
2023-09-19